Webhooks are useful in cases where a specific code needs to be executed when an event occurs. For example, we can set a webhook to trigger an endpoint when confirming purchase order, creating a new product, sell a product through pos, etc. so, instead of having to check each minute to see if there is a new event happening or not, we can tell the app that this event has occurred in odoo using webhooks. This helps to reduce the number of requests sent. We can store the data as json or xml in a webhook.
Let us see how to send a webhook using a simple python example. For this, first, we need to import the required libraries which are requests and json.
import requests
import json
requests here is used for sending the http request. json is for formatting the payload. Next, we need a URL for the webhook.
webhook_url = 'http://127.0.0.1:5000/webhook'
Now we need some data that need to be sent to the URL
data = { 'name': 'This is an example for webhook' }
Now we need to generate the request. Here we are using the post method to send the http request. We specify the parameters which are the URL, data formatted as json, and in headers we specify the content type.
requests.post(webhook_url, data=json.dumps(data), headers={'Content-Type': 'application/json'})
Instead of using a local url, we can also try testing the request on a site like the webhook site. We can get a unique url from there for testing. When we go to the website, we can see the data that we send in there.
import requests
import json
webhook_url = 'https://webhook.site/fbca32a8-1303-4ba4-868c-db6ed772c5fc'
data = { 'name': 'This is an example for webhook' }
requests.post(webhook_url, data=json.dumps(data), headers={'Content-Type': 'application/json'})
It will show the data that we send to the url as below
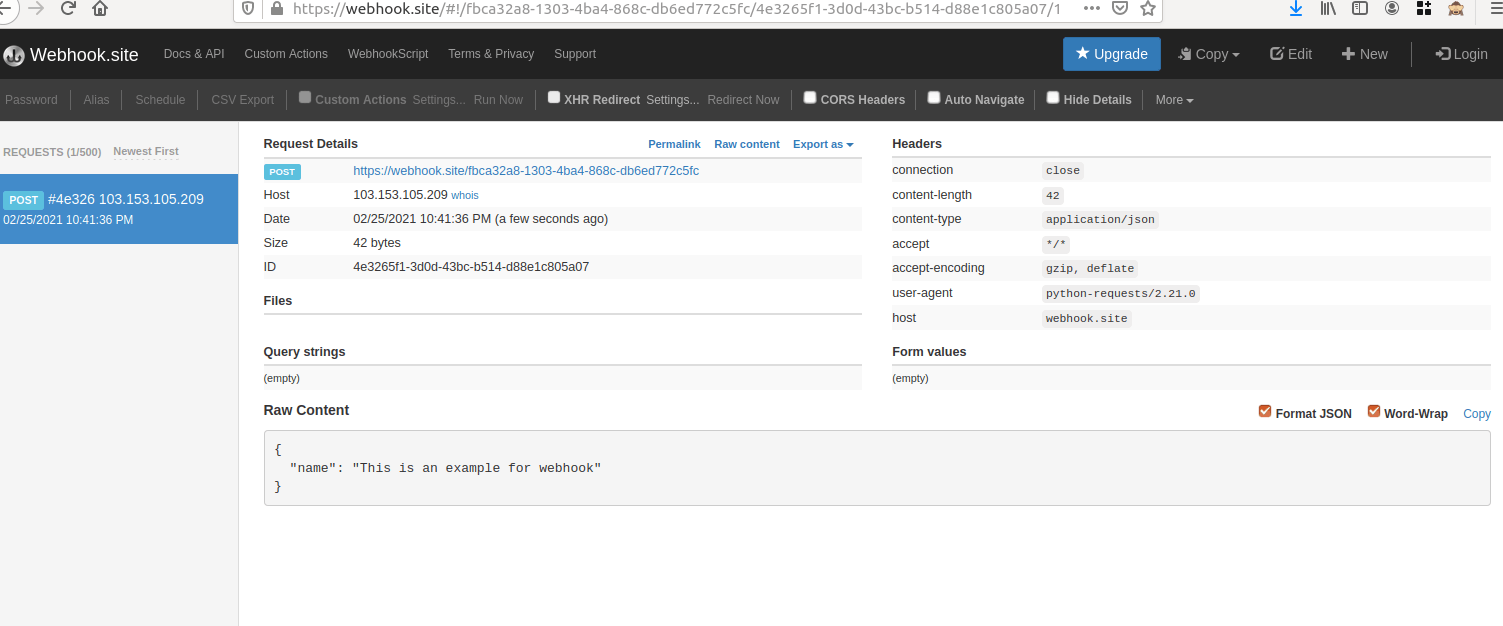
Now let’s see how to receive a webhook using python.
So we will create a route that listens to webhooks. Let's use the micro-framework flask for this example. If the flask is not already installed, install it from the terminal as below.
pip install flask
Now that flask is installed, let’s import the required libraries for our example which are flask, request, and abort.
Let’s add the part of url that the server will listen to for this webhook in the route section. So for example, whenever we go to the url ‘http://127.0.0.1:5000/webhook the function with this route will be executed.
@app.route('/webhook', methods=['POST'])
So now let us define the function to be executed whenever a post request is received in this url.
def get_webhook():
if request.method == 'POST':
print("received data: ", request.json)
return 'success', 200
else:
abort(400)
So if a get request is made, then else part will work and abort with status 400. If a post request is made, then it will print the json data that we received and then return message success with status 200. Now we just add all these codes to a file and run it from the terminal.
from flask import Flask, request, abort
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def get_webhook():
if request.method == 'POST':
print("received data: ", request.json)
return 'success', 200
else:
abort(400)
if __name__ == '__main__':
app.run()
I have saved this file as server.py and in terminal, we will run it as python3 server.py.
When we run this code in the terminal, we can see the address at which it is running the server.
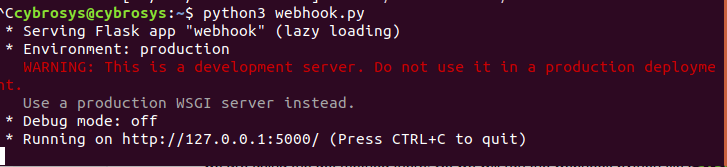
Here we can see the url at which it is running is http://127.0.0.1:5000/. And to get our webhook called, we go to url http://127.0.0.1:5000/webhook. When we go to this url through chrome, the else condition will work and show ‘Method Not Allowed’ since we are using the get method there. So we will run the webhook.py that we created first for sending requests with website_url as http://127.0.0.1:5000/webhook. For this, we run the command ‘python3 server.py’ in the terminal. Now we can see that the received data at the function with route ‘/webhook’ contains the data that we send via the post method.
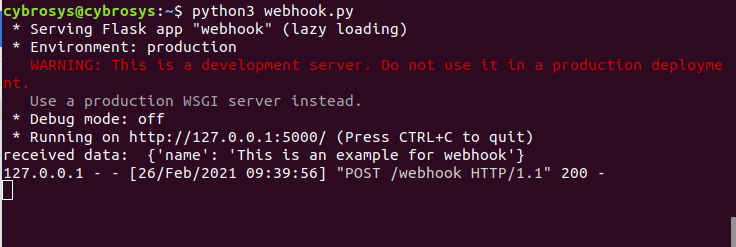