JavaScript is a Synchronous, single-threaded, and non-blocking language.
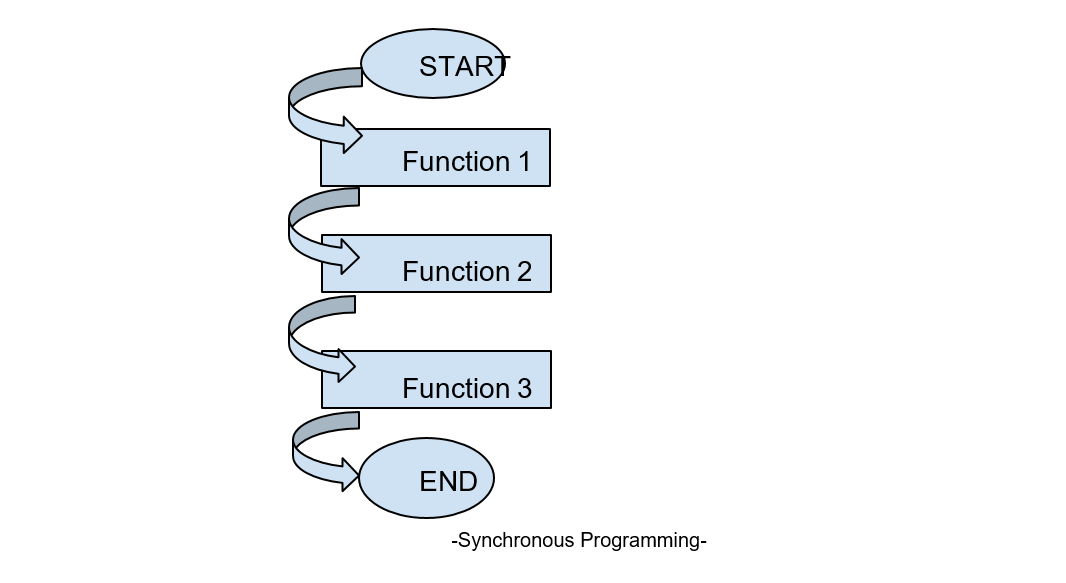
JavaScript is a Synchronous language which means that every function is done in a sequence. Each action must wait for the completion of the previous action. When the Js calls a function, it will add to the stack, and the execution will start. By default, the programming is working synchronously. This is time-consuming, so to overcome that, the asynchronous methods are used in javascript.
Example:
function test1(){
console.log('test1');
};
function test2(){
console.log('test2');
};
function test3(){
console.log('test3');
};
test1()
test2()
test3()
Output:
"test1"
"test2"
"test3"
? The setTimeout() and The setInterval()
? JavaScript Callbacks
? JavaScript Promise
? javascript async/await
The setTimeout() and The setInterval()
setTimeout:
The setTimeout() method invoke a function after the specified time. The setTimeout() method executes only once.
General syntax:
setTimeout(function, duration, param1, param2, param3,.....)
Example with the basic syntax:
function test1(){
console.log('Hi, How are you');}
setTimeout(test1,5000)
Logs: After 5 seconds display "Hi, How are you" to the console
setInterval()
The setInterval() method invokes a function at specified intervals. Repeatedly runs the same codes at regular intervals over and over again.
General syntax:
setInterval(function, duration, param1, param2, param3,.....)
Example with the basic syntax:
function test1(){
console.log('Good morning');}
setInterval(test1,3000)
Logs: "Good morning" every two seconds in the console.
JavaScript Callbacks
Callback in javascript means a function can be passed as an argument to another function. And that executes based on the result.
Example with the basic syntax:
function testCase(example) {
document.getElementById("hello").innerHTML = example;}
function simpleCalculator(number1, number2, simpleCallback) {
let result = number1 + number2;
simpleCallback(result);}
simpleCalculator(3, 3, testCase);
Logs: 6 is in the console
This is a simple example for callbacks in js, In this example, testCase is the name of the function. This is passed to another function simpleCalculator(), as an argument.
Javascript promises
A Promise in JavaScript is a simple object that links producing code and consuming code. Promises are the methods used to handle asynchronous operations in JavaScript.
Always a promise object in any of the three states:
? Pending
? Fulfilled
? Rejected
Pending: which is working, the result is undefined.
Fulfilled: Operation completed successfully; the result is a value.
Rejected: Operation failed; the result is an error value.
Example with the basic syntax:
let x = new Promise((newResolve,newReject) =>{
let sum = 2+2
if(sum==4){
newResolve('Correct')}
else{
newReject('Incorrect')}
})
x.then((message)=>{
console.log('then is this'+message)
}).catch((message)=>{
console.log('catch is this'+message)})
Logs:"then is this correct" in the console
Because here the condition is correct so in the console - "then is this correct"
Example with the basic syntax:
let x = new Promise((newResolve,newReject) =>{
let sum = 2+3
if(sum==4){
newResolve('Correct')}
else{
newReject('Incorrect')}
})
x.then((message)=>{
console.log('then is this'+message)
}).catch((message)=>{
console.log('catch is this'+message)
})
Logs: "catch is this Incorrect" in the console.
Because here the condition is Incorrect so in the console - "catch is this Incorrect"
Both then and catch methods return promises.
javascript async/await
The async and await are the keyword that is used to define an asynchronous action in javascript programming.
async function newFunction() {
return "How are you?";}
newFunction().then(
function(correct) {newDisplayer(correct);},
function(incorrect) {newDisplayer(incorrect);});
Logs: “How are you?” displayed to the console.
The await is also a keyword that is used inside the async function, which waits for the promise and gets a fulfilled response. The await will return the result of the action after the asynchronous action completes,
Example with the basic syntax:
async function newExample() {
let newPromise = new Promise(function(resolve, reject) {
resolve("I'am Fine");
});
document.getElementById("test").innerHTML = await newPromise;}
newExample();
Logs: “I’am Fine display on the console
The list above includes the most used asynchronous methods and their applications. To understand more about each feature, try it out.