Array is a built-in global object that allows us to store multiple elements at once, not only in Javascript but also in other programming languages also. In this blog, we’ll be discussing the different inbuilt functions used in Javascript to perform operations in arrays.
1. concat()
The concat method is useful when you need to merge two or more than two arrays.
Syntax:
array_1.concat(array_2, array_3,..., array_N)
Eg:
var array_1 = ["apple", "orange", "watermelon"]
var array_2 = ["grape", "mango"]
console.log(array_1.concat(array_2))
The console will show an array of 5 elements which is the combination of array_1 and array_2.
2. copyWithin()
The copyWithin method is used to copy part of the array to another location.
Syntax:
array_1.copyWithin(target[start], target[end])
Eg:
var array_1 = ["apple", "orange", "watermelon"]
console.log(array_1.copyWithin(2,0))
The console will show an array with elements [“apple”, “orange”, “apple”] as the element in position 2 is replaced by the element in 0th position.
3. entries()
The entries method is used as an array iterator with key/value pairs for each index. It means that a new array is created that contains the key and value pairs for each index of an array.
Syntax:
array_1.entries()
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
for (let x of array_1.entries()) {
console.log(x)
}
Consoling this will give us the position and value of the element in array format.
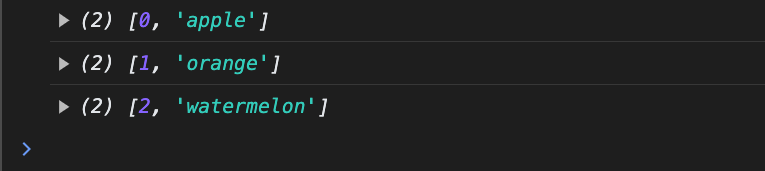
4. every()
To check if all elements in the array pass the test, every method is used. It executes a function for each array element and returns true if the function returns true for all elements in the array or false if the function returns false for all elements in the array.
Syntax:
array_1.every(function)
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
console.log(array_1.every(startsWithA))
function startsWithA(elt) {
return elt.startsWith('a')
}
The console will return ‘false’ as the condition is not satisfied for all elements.
5. fill()
To change the elements in an array to static value, the fill method is used. It fills specified elements in an array with a value.
Syntax:
array_1.fill(value, start_index, end_index)
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
array_1.fill("kiwi")
console.log(array_1)
Consoling this would return the array with all elements as “kiwi”. If we give the position as arguments, then only those elements will be filled.
6. filter()
The filter method can be used to create a new array with the elements that pass the test which are provided by a function. The original array is not changed when using the filter method.
Syntax:
array.filter(function(currentValue, index, arr), thisValue)
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
console.log(array_1.filter(startsWithA))
function startsWithA(elt) {
return elt.startsWith('a')
}
Consoling this will give the array of elements that start with the letter a.
7. find()
To return the value of the first element that matches the test, the find method is used. The find method executes a function for each array element. It returns the value if the function is matched and returns undefined if no elements are found.
Syntax:
array.find(function(currentValue, index, arr),thisValue)
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
console.log(array_1.find(startsWithA))
function startsWithA(elt) {
return elt.startsWith('a')
}
In this, the console will give ‘apple’ as the apple is the only element that starts with ‘a’.
8. findIndex()
The findIndex method returns the index of the first element that matches the test. It returns -1 if no match is found.
Syntax:
array.findIndex(function(currentValue, index, arr), thisValue)
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
console.log(array_1.findIndex(startsWithA))
function startsWithA(elt) {
return elt.startsWith('a')
}
Here, the console gives 0, which is the index of the element which starts with ‘a’, ‘apple’.
9. flat()
The flat() method concatenates sub-array elements.
Syntax:
array.flat(depth)
Eg:
var array_1 = [['apple', 'orange'], 'watermelon', ['blueberry', 'blackberry']]
console.log(array_1.flat())
The console here gives an array with the elements inside the main array. The sub-array elements are concatenated using the flat() method.

10. flatMap()
The flatMap() method is used to map all array elements in the array and create a new flat array that satisfies the conditions passed by the flatmap method.
Syntax:
array_1.flatMap(condition);
Eg:
var array_1 = [1, 2, 3, 4, 5,6];
var array_2 = array_1.flatMap((x) => x * 2);
console.log(array_2)
The console gives a new array which is formed by multiplying the elements of the first array with 2.
11. forEach()
To call a function for each element in the array, the forEach method is used. This method does not call for empty elements in the array.
Syntax:
array_1.forEach(myFunction);
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
array_1.forEach(forEachFn)
function forEachFn(elt) {
console.log(elt)
}
The console will give us the elements in array_1.
12. includes()
If the element is present in the array, the includes method returns True else it will return False.
Syntax:
array_1.includes("value");
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
console.log(array_1.includes('orange'))
The console gives true as orange is present in array_1.
13. indexOf()
The indexOf() method is used to find the index of an element of an array. This method returns the index of the element if it's present in the array. Else, returns -1.
Syntax:
array.indexOf(‘value’)
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
console.log(array_1.indexOf('watermelon'))
The console will give 2, which is the index value of watermelon in array_1
14. join()
The join() method returns the array as a string. You can pass text as an argument to add text when joining the elements in the array.
Syntax:
array.join(‘value’)
Eg:
var array_1 = ['apple', 'orange', 'watermelon']
console.log(array_1.join(' and '))
The console will give the result as

The above-mentioned methods are the different inbuilt functions used in Javascript to perform operations in arrays. Make use of these methods wisely for optimizing your code and for better performance.
To read more about the QWeb template in Odoo 16, refer to our blog What is QWeb template in Odoo 16