A built-in module in Python, the base64 library offers functions for encoding and decoding binary data to and from base64 strings, effectively converting any binary data to plain text. A common encoding method called Base64 converts binary data into a string of printable ASCII characters. This enables the secure transmission of binary data via protocols like email and HTTP that only handle ASCII characters.
The base64 module provides two interfaces for encoding and decoding binary data:
* The modern interface: This interface allows you to encode things that resemble bytes in ASCII bytes and decode items that resemble bytes or strings that contain ASCII in bytes.
* The legacy interface: It offers operations for encoding and decoding to and from file objects but does not provide decoding from strings.
For the majority of apps, the modern interface is recommended. Compared to the legacy interface, it is more adaptable and effective.
You must first import the base64 module into your Python programme before you can use it. After importing the module, you can encode and decode data using the following functions:
* b64encode(): This function encodes a bytes-like object to ASCII bytes using the specified encoding.
* b64decode(): This function decodes an ASCII bytes object to bytes using the specified encoding
It supports the following encodings:
* Base16: This encoding uses two hexadecimal digits to represent each byte of data.
* Base32: This encoding uses five ASCII digits to represent each byte of data.
* Base64: This encoding uses four ASCII characters to represent each byte of data.
* Ascii85: This encoding uses eight ASCII characters to represent each three bytes of data.
* Base85: This encoding uses eight ASCII characters to represent each four bytes of data.
Base64 encoding:
Each group of three bytes is stored as a group of four characters from the following set to encode data:
* ABCDEFGHIJKLMNOPQRSTUVWXYZ (26 uppercase letters)
* Abcdefghijklmnopqrstuvwxyz (26 lowercase letters)
* 0123456789 (10 numbers)
* + and / for new lines
Additionally, the data stream is padded at the end with the = character.
Six bits of data are represented by each Base64 character. The procedures listed below must be taken in order to convert a string into a Base64 character:
* Get the ASCII value of each character in the string.
* Compute the 8-bit binary equivalent of the ASCII values
* By reorganising the digits, divide the 8-bit character chunk into 6 bit pieces.
* Convert the 6-bit binary groups to their respective decimal values.
* Align the corresponding Base64 values for each decimal number using the Base64 encoding table..
An illustration of a Base64 encoding table is shown below.
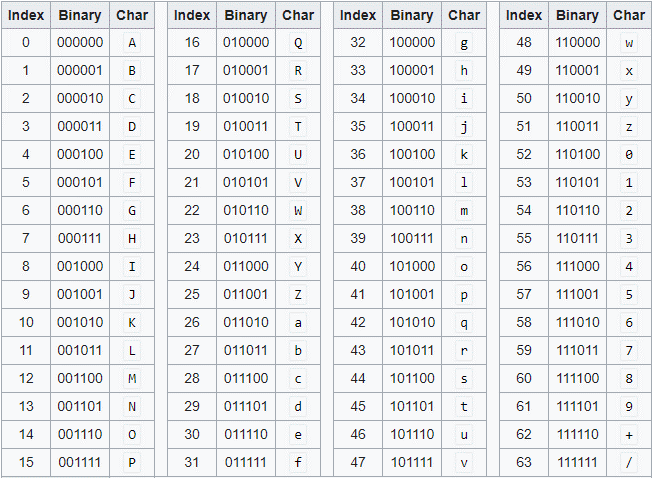
The `base64` library provides several functions to encode data:
1.`base64.b64encode(data)`: Encodes the given `data` (bytes-like object) and returns the encoded data as a bytes object.
2.`base64.b64encode(data).decode()`: Encodes the data and returns the encoded data as a string (Unicode).
3.`base64.b64encode(data, altchars=None)`: Encodes the data using the specified alternative characters, if provided.
An example of the encoding of a string :
import base64
data = "Python is a programming language"
data_bytes = data.encode('ascii')
base64_bytes = base64.b64encode(data_bytes)
print("Encoded Data: ", base64_string)
# Output:
EncodedData: UHl0aG9uIGlzIGEgcHJvZ3JhbW1pbmcgbGFuZ3VhZ2U=
base64.encodestring() This technique allows us to directly encode the string. We must import 'encodestring' from the base64 library in order to use this method.
Example:
import base64
from base64 import encodestring
string = ‘Python is a programming language’
encode_data = encodestring(string)
print(“encode_data:”, encode_data)
# Output:
encode_data: UHl0aG9uIGlzIGEgcHJvZ3JhbW1pbmcgbGFuZ3VhZ2U=
Example of Encode Files:
import base64
message = "life of brian"
file = open("python.txt", "w")
file.write(MESSAGE)
file.close()
base64.encode(open("python.txt"), open("python.b64", "w"))
print "encoded message:", repr(open("out.b64").read())
# Output:
encoded message: 'bGlmZSBvZiBicmlhbg==\012'
Base64 decoding:
The `base64` library provides functions to decode Base64-encoded data:
1. base64.b64decode(encoded_data`: Decodes the Base64-encoded `encoded_data` (bytes-like object) and returns the decoded data as a bytes object.
2. base64.b64decode(encoded_data.encode()): Decodes the encoded data (as a string) and returns the decoded data as a bytes object.
3. base64.b64decode(encoded_data, altchars=None): Decodes the encoded data using the specified alternative characters, if provided.
An example of the encoding of a string :
import base64
data = "UHl0aG9uIGlzIGEgcHJvZ3JhbW1pbmcgbGFuZ3VhZ2U="
data_bytes = data.encode('ascii')
base64_bytes = base64.b64encode(data_bytes)
base64_string = base64_bytes.decode('ascii')
print("Decoded Data: ", base64_string)
# Output:
Decoded Data: Python is a programming language
Syntax of other base64 encoding and decoding methods:
* base64.b64encode(s, altchars=None)
* base64.b64decode(s, altchars=None, validate=False)
* base64.standard_b64encode(s)
* base64.standard_b64decode(s)
* base64.urlsafe_b64encode(s)
* base64.urlsafe_b64decode(s)
* base64.b32encode(s)
* base64.b32decode(s)
* base64.b16encode(s)
* base64.b16decode(s)
* base64.a85encode(b, foldspaces=False, wrapcol=0, pad=False, adobe=False)
* base64.a85decode(b, foldpaces=False, adobe=False, ignorechars=b'\t\n\r\v')
* base64.b85encode(b, pad=False)
* base64.b85decode(b)
Parameters:
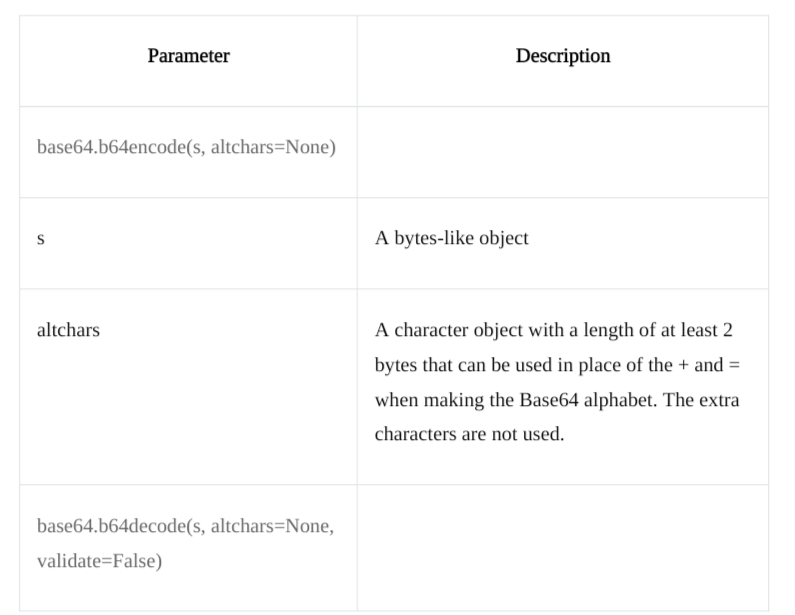
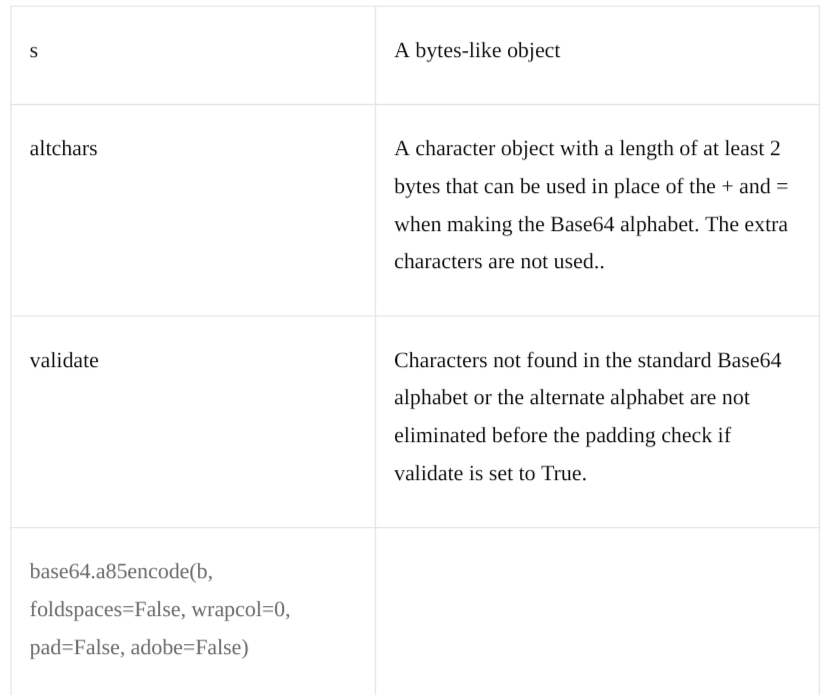
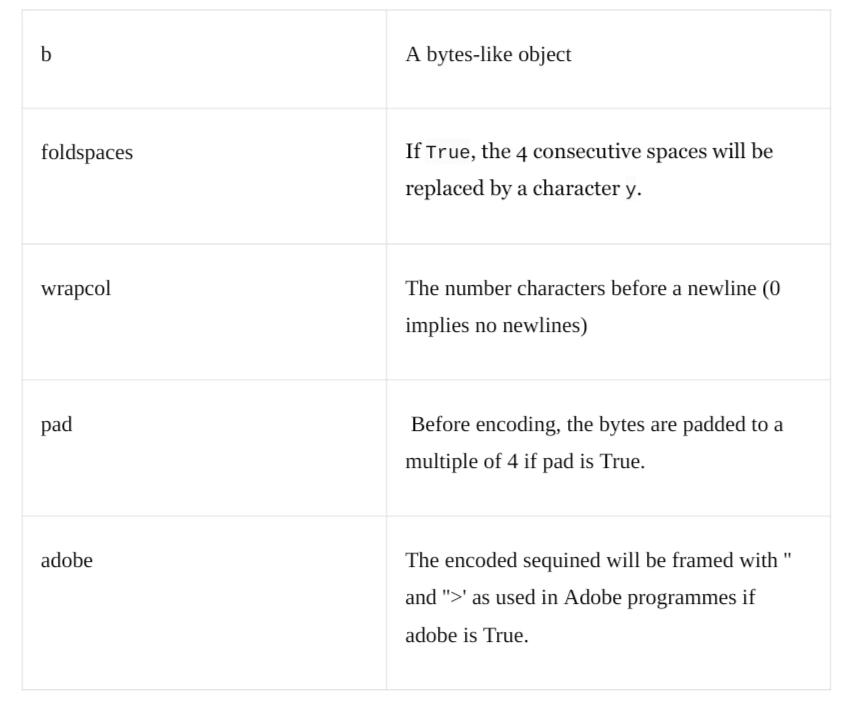
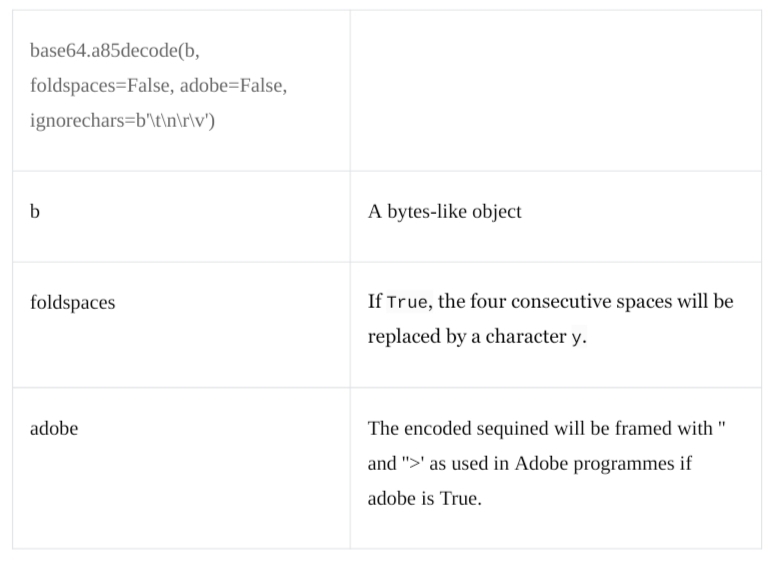
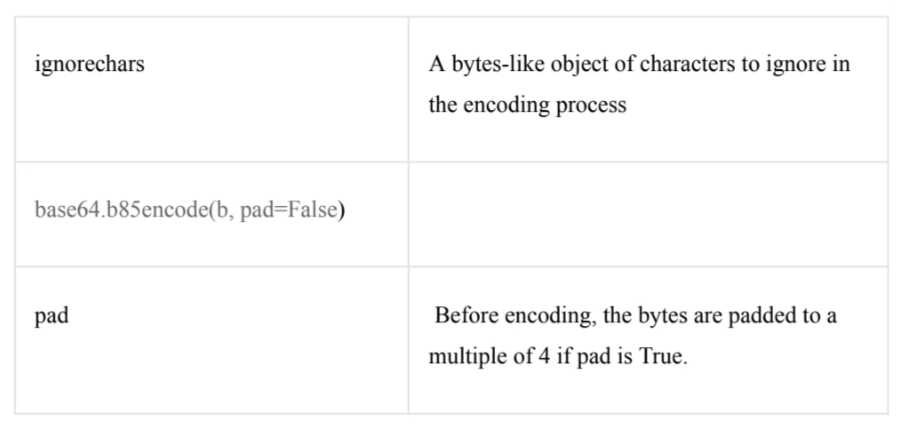
Other key details of base64 python library
* URL-safe encoding and decoding:
The base64 library also supports URL-safe encoding and decoding using functions like `base64.urlsafe_b64encode()` and `base64.urlsafe_b64decode()`. These functions use a modified Base64 alphabet that is safe for use in URLs.
* Padding and non-padding variants:
By default, the base64 library uses the standard Base64 encoding with padding (using "=" characters to make the encoded data a multiple of 4 bytes in length). However, the library also provides non-padding variants for encoding and decoding, such as base64.b64encode() and base64.b64decode(), respectively.
* Handling errors:
The base64 library raises `binascii.Error` if the input data is incorrectly padded or contains non-alphabet characters during decoding.
The Python base64 module provides an easy way to encrypt and decrypt binary data using the Base64 encoding technique. It is also extremely simple to use. It is frequently employed for purposes like encoding binary files for email transmission or including binary data in JSON or XML payloads.