Python's num2words module, which translates numbers (like 37) to words (like thirty-seven). This library offers support for other languages as well. In this blog we can see how to translate numbers to words using the num2words package.
Installation of num2words
Num2words can be easily installed via pip:
pip install num2words
For the pip3 version,
Pip3 install num2words
If the package is already installed you get an output like this,

Otherwise, the source package can be downloaded and then executed:
python setup.py install
Usage
There is only one function to use- import the num2words package.
from num2words import num2words
Convert number
text = num2words(value, lang='en_IN')
Besides the numerical argument, there are two optional arguments.
value: Boolean flag indicating the return of an ordinal value instead of a cardinal one.
lang: The language in which the number is to be converted. Example for the supported values are:
- en (English, default)
- en-IN (Indian English)
- en-GB (British English)
- fr (French)
- lt (Lithuanian)
- de (German)
- es (Spanish)
- lv (Latvian)
You can supply values like fr_FR, the code will be interpreted correctly. If you provide an unsupported language, NotImplementedError will be raised. So if you want to call num2words with a fallback, you can do the following:
try:
return num2words(42, lang=’my language’)
except NotImplementedError:
return num2words(42, lang='en')
Here, I will give an example for using num2words in Odoo, This will allow you to convert invoice amount to text. You can choose the language from the account setting.
account setting:
*.py file
from odoo import fields, models,api, _
class CompanyLanguage(models.Model):
_inherit = "res.company"
text_amount_language_currency = fields.Selection([('en', 'English'),
('ar', 'Arabic'),
('cz ', 'Czech'),
('de', 'German'),
('dk', 'Danish'),
('en_GB', 'English - Great Britain'),
('en_IN', 'English - India'),
('es', 'Spanish'),
('es_CO', 'Spanish - Colombia'),
('es_VE', 'Spanish - Venezuela'),
('eu', 'EURO'),
('fi', 'Finnish'),
('fr', 'French'),
('fr_CH', 'French - Switzerland'),
('fr_BE', 'French - Belgium'),
('fr_DZ', 'French - Algeria'),
('he', 'Hebrew'),
('id', 'Indonesian'),
('it', 'Italian'),
('ja', 'Japanese'),
('ko', 'Korean'),
('lt', 'Lithuanian'),
('lv', 'Latvian'),
('no', 'Norwegian'),
('pl', 'Polish'),
('pt', 'Portuguese'),
('pt_BR', 'Portuguese - Brazilian'),
('sl', 'Slovene'),
('sr', 'Serbian'),
('ro', 'Romanian'),
('ru', 'Russian'),
('sl', 'Slovene'),
('tr', 'Turkish'),
('th', 'Thai'),
('vi', 'Vietnamese'),
('nl', 'Dutch'),
('uk', 'Ukrainian'),
], string='Text amount language/currency')
class AccountConfigSettings(models.TransientModel):
_inherit = 'res.config.settings'
text_amount_language_currency = fields.Selection(related="company_id.text_amount_language_currency",
string='language_currency', readonly=False)
@api.onchange('text_amount_language_currency')
def save_text_amount_language_currency(self):
if self.text_amount_language_currency:
self.company_id.text_amount_language_currency = self.text_amount_language_currency
*.xml file
<odoo>
<record id="num_to_words_inherit_view_account_config_settings" model="ir.ui.view">
<field name="name">Add num to words configuration</field>
<field name="model">res.config.settings</field>
<field name="inherit_id" ref="base.res_config_settings_view_form"/>
<field name="arch" type="xml">
<xpath expr="//div[hasclass('settings')]" position="inside">
<div class="app_settings_block">
<h2>Text amount</h2>
<div class="row mt16 o_settings_container">
<div class="col-xs-12 col-md-6 o_setting_box">
<div class="o_setting_left_pane"/>
<div class="o_setting_right_pane">
<div class="content-group">
<div class="row mt16">
<label for="text_amount_language_currency" string="Language / currency" class="col-lg-3 o_light_label"/>
<field name="text_amount_language_currency" readonly="0" widget="selection"/>
</div>
</div>
</div>
</div>
</div>
</div>
</xpath>
</field>
</record>
</odoo>
Invoice form view
*.py
from num2words import num2words
from odoo import fields,api, models, _
class InvoiceText(models.Model):
_inherit = "account.move"
text_amount = fields.Char(string="Total In Words", required=False, compute="amount_to_words" )
@api.depends('amount_total')
def amount_to_words(self):
if self.company_id.text_amount_language_currency:
self.text_amount = num2words(self.amount_total, to='currency',
lang=self.company_id.text_amount_language_currency)
*.xml
<odoo>
<record id="add_text_amount_to_invoice_form" model="ir.ui.view">
<field name="name">add.text.amount.to.invoice.form</field>
<field name="model">account.move</field>
<field name="inherit_id" ref="account.view_move_form"/>
<field name="arch" type="xml">
<xpath expr="//sheet/group/group/field[@name='invoice_date']" position="after">
<field name="text_amount"/>
</xpath>
</field>
</record>
</odoo>
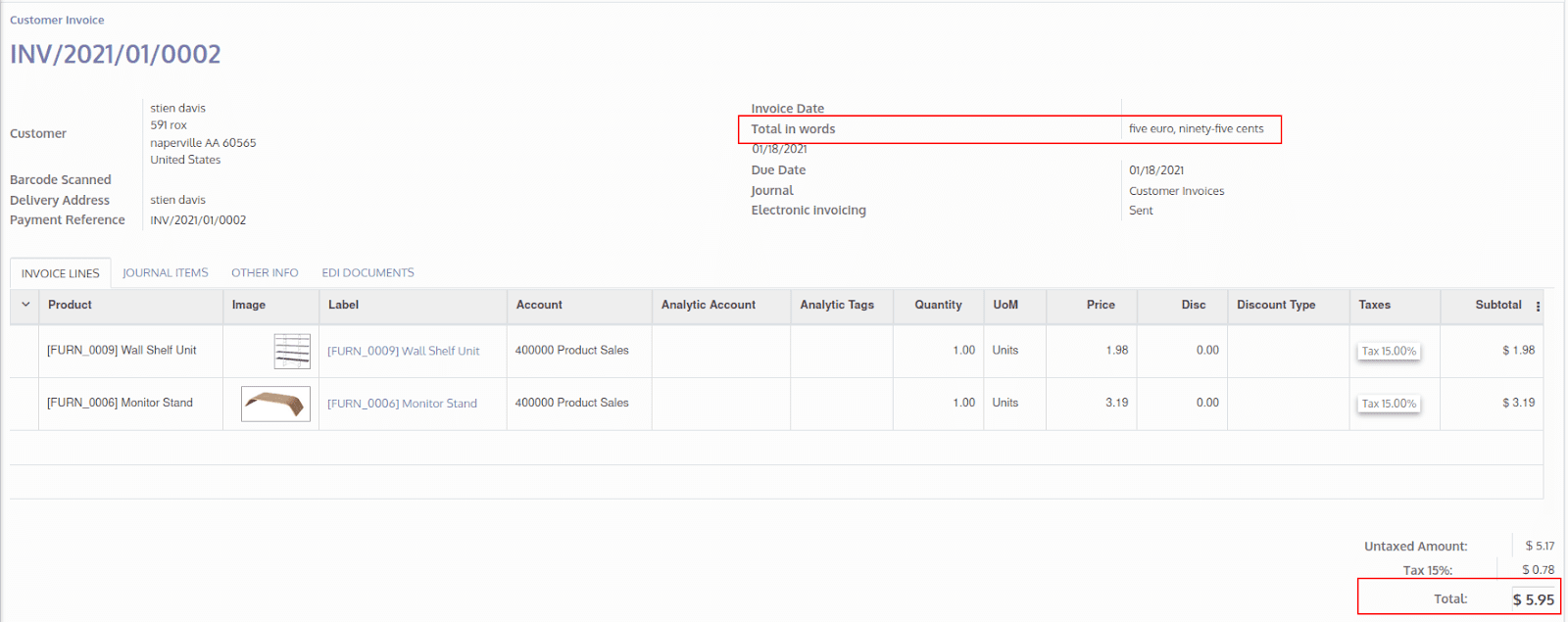
If you choose the Language as Arabic in Accounting -> config -> settings
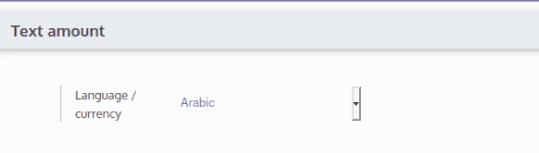
The Total will be shown in Arabic words, ie, the Arabic number name will be shown there.