AJAX (Asynchronous JavaScript and XML) used to create interactive, dynamic web pages. Ajax loads data from the server and updates the browser without reloading the entire page.
Now we can check how ajax is implemented in Odoo 16.
Code-block::javascript
odoo.define('module.Something', function (require) {
"use strict";
var ajax = require('web.ajax');
return ajax.jsonRpc(...).then(function (result) {
// some code here
return something;
});
});
Here, we will load some data by an RPC and simply return a promise by the module. Then, before registering the module, it will simply wait for the promise. This code defines a module named 'module.something'. This module uses the ’web.ajax’ module to make an asynchronous call to the server using JSON-RPC. The result of the server call is processed within a callback function defined using the.then() method of the promise returned by the server call.
For example, a corresponding vehicle will be shown as we choose a customer in the Subscription cancellation form on the website.
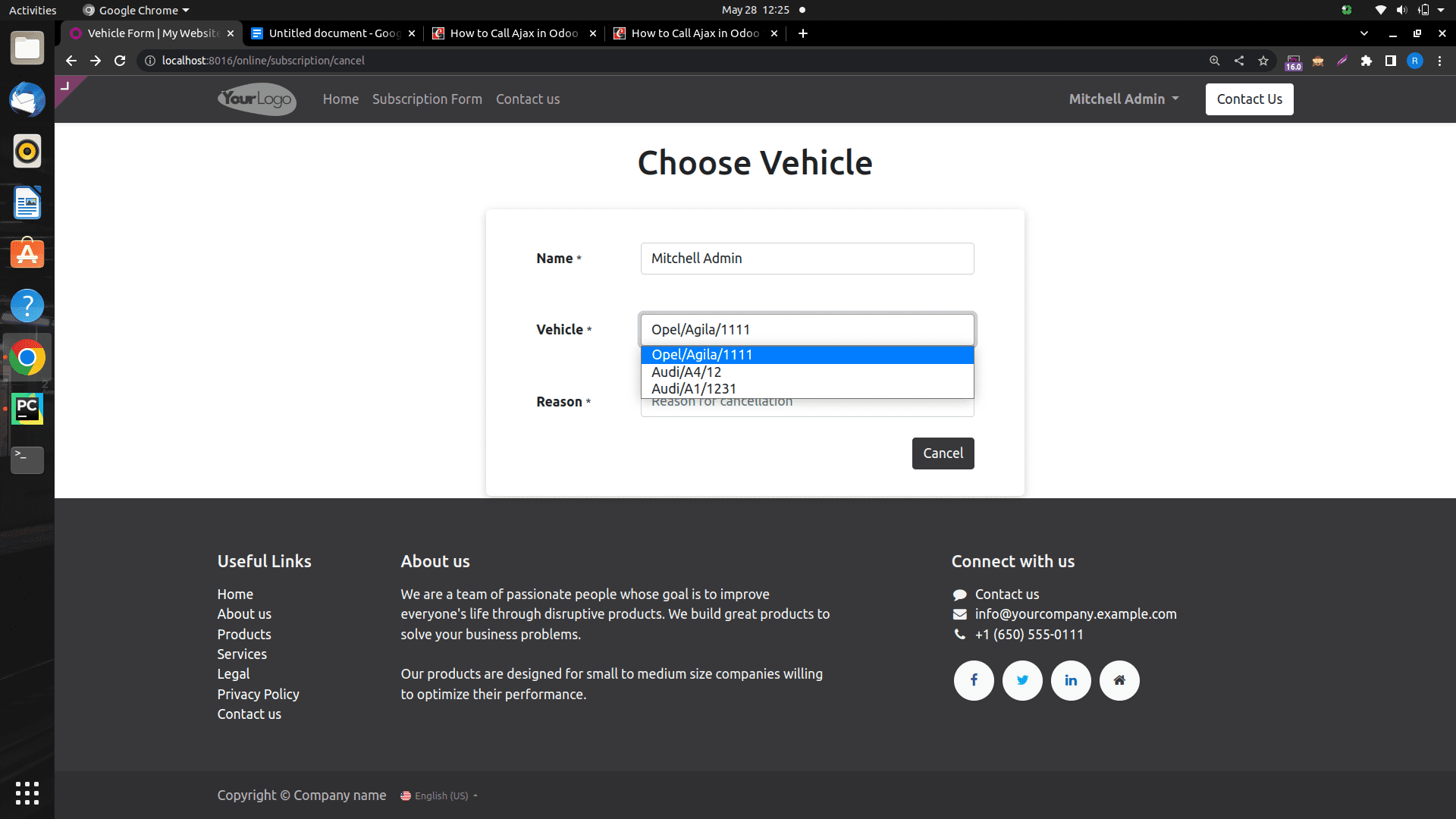
For this, first we need a template for subscription cancellation, as shown below.
<template id="subscription_cancellation_form"
name="Vehicle Form">
<t t-call="website.layout">
<div id="wrap" class="oe_structure cancel_sub">
<section class="s_website_form" id="book_my_vehicle" data-vcss="001" data-snippet="s_website_form">
<div class="container">
<h1 style="text-align: center;">Choose Vehicle</h1>
<form action="/online/cancellation/click" method="post" enctype="multipart/form-data"class="o_mark_required" data-mark="*" data-model_name="" data-success-page="">
<div class="s_website_form_rows row s_col_no_bgcolor">
<div class="form-group col-12 s_website_form_field
s_website_form_required"data-type="char"
data-name="Field">
<div class="row s_col_no_resize s_col_no_bgcolor">
<label class="col-form-label col-sm-auto
s_website_form_label" <span class="s_website_form_label_content">Name</span>
<span class="s_website_form_mark">*</span>
</label>
<div class="col-sm">
<div class="col-sm">
<input class="form-control s_website_form_input" id="customer_name"name='customer' type="text"/>
</div></div></div>
<div class="row s_col_no_resize s_col_no_bgcolor">
<label class="col-form-label col-sm-auto s_website_form_label”>
<span class="s_website_form_label">Vehicle</span>
<span class="s_website_form_mark">*</span></label>
<div class="col-sm">
<select id="vehicle_cancellation" type="text"class="form-control s_website_form_input"
name="vehicle" required="1">
<option t-att-value=""/>
<t t-foreach="vehicles or []" t-as="vehicle">
<option t-att-value="vehicle.id" required="1">
<t t-esc="vehicle.name"></t>
</option></t>/select></div></div>
<div class="row s_col_no_resize s_col_no_bgcolor">
<label class="col-form-label col-sm-auto s_website_form_label">
<span class="s_website_form_label">Reason</span>
<span class="s_website_form_mark">*</span>
</label>
div class="col-sm">
<input id="reason" type="text" class="form-control s_website_form_input"name="reason" required="1" placeholder="Reason for cancellation"/>
</div></div>
<div class="form-group col-12 s_website_form_submit" data-name="cancel Button">
<div style="width:200px;"class="s_website_form_label"/>
<button type="submit" id="cancel" class="btn btn-primary" style="float: right;">Cancel</button>
</div></form></div></section></div></t></template>
Next we can call Ajax from the Change function in the js file.Here inside onChangeCustomer function Ajax call takes place.
_onChangeCustomer: async function(ev){
var customer_id = $('input[name="customer"]')[0].value
await ajax.jsonRpc('/online/choose/vehicle', "call",
{'customer_id': customer_id})
.then(function(result) {
const select=document.
querySelector('#vehicle_cancellation');
const options = Array.from(select.options);
options.forEach((option) => {
option.remove();
});
result.forEach((item) => {
let newOption = Option(item[1],item[0]);
select.add(newOption, undefined);
});
});
}
For this, we need to include web.ajax in js file that is, const ajax =require(web.ajax);
Using jsonRpc, it makes AJAX request. The request is sent to the URL, and the method called is “call.”The URL handles JSON RPC request. This is written in the py file, as shown below. await ajax.jsonRpc('/online/choose/vehicle', "call", {'customer_id': customer_id}):This line uses the ajax.jsonRpc() method from the web.ajax module to make an asynchronous JSON-RPC call to the server. The first argument is the URL endpoint for the server call, which is '/online/choose/vehicle' in this case. The second argument is the JSON-RPC method name, which is "call" here. The third argument is an object containing the parameters to be sent to the server. In this case, it includes a single parameter, 'customer_id', with the value retrieved earlier.
.then(function(result) { ... }): This is a callback function that executes when the Promise returned by ajax.jsonRpc() is resolved (i.e., when the server responds). It takes the result parameter, which represents the response received from the server.
@http.route('/online/choose/vehicle', type='json', auth="public", website=True)
def choose_vehicle(self, **kwargs):
customer = kwargs.get('customer_id')
customer_id = request.env['res.partner'].sudo()
.search([('name', '=', customer)])
vehicle_id =request.env['fleet.subscription'].sudo()
.search([('state', '=', 'subscribed'), ('customer_id', '=',customer_id.id)]).mapped('vehicle_id')
vehicle = []
if vehicle_id:
for rec in vehicle_id:
vehicle.append((rec.id, rec.name))
return [*set(vehicle)]
Here it sends a request to the server with the customer id, and it updates the option of select element with the received data which is returned from here.
@http.route('/online/choose/vehicle', type='json', auth="public", website=True).
This decorator defines the route URL for the Ajax request. The Ajax request should be made to '/online/choose/vehicle'.type='json' specifies that the response will be in JSON format.auth="public" allows the route to be accessible without authentication.
Website=True indicates that the route is part of the website module.