What is a math object in JavaScript?
Math object is a built-in static object with properties and methods for mathematical constants and functions. It is used to perform complex math operations. Math is not a constructor.
Math Properties (Constants):
Syntax: Math.property
The math object properties are;
1. Math.E: It represents the Euler's number and the base of natural logarithms.
2. Math.PI: This property represents the ratio of the circumference of a circle to its diameter, approximately 3.1415
3. Math.SQRT2: Square root of 2; approximately 1.414.
4. Math.SQRT1_2: Square root of ½; approximately 0.707.
5. Math.LN2: Natural logarithm of 2, approximately 0.693.
6. Math.LN10: Natural logarithm of 10; approximately 2.303.
7. Math.LOG2E: Base-2 logarithm of E; approximately 1.443.
Example:
<!DOCTYPE html>
<html>
<head>
<title> JavaScript Math Object </title>
</head>
<body>
<h2>JavaScript Math Properties</h2>
<p id="demo"></p>
<!-- Script to return math property values -->
<script>
document.getElementById("demo").innerHTML =
"<p><b>Math.E: </b>" + Math.E + "<br></p>" +
"<p><b>Math.PI: </b>" + Math.PI + "<br></p>" +
"<p><b>Math.SQRT2: </b> " + Math.SQRT2 + "<br></p>" +
"<p><b>Math.SQRT1_2: </b> " + Math.SQRT1_2 +
"<br></p>" +
"<p><b>Math.LN2: </b>" + Math.LN2 + "<br></p>" +
"<p><b>Math.LN10: </b>" + Math.LN10 + "<br></p>" +
"<b>Math.LOG2E: </b>" + Math.LOG2E;
</script>
</body>
</html>
Save the file with the .html extension. Then open it in any browser like Chrome, or Firefox. It will give the output as;
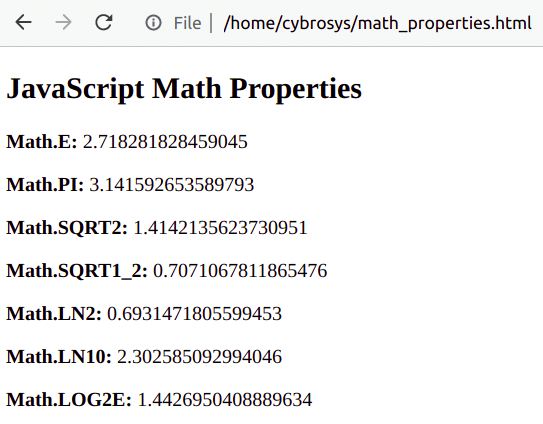
Math Methods:
Syntax: Math.method(number)
There are many math methods. They are;
1. Math.abs(x) It will return the absolute value (+ve) of the given value.
2. Math.sin(x) It will return the sine of a given angle in radians.
3. Math.cos(x) It will return the cosine of a given angle in radians.
4. Math.tan(x) Returns the tangent of a number.
5. Math.min(a, b, …) Returns the smallest number passed in the method.
6. Math.max(a, b, …) This method returns the largest value of the given numbers.
7. Math.pow(x, y) Returns the result of a value 1 (base) raise to the power of value 2 (exponent)
8. Math.exp(x) It will return Ex (Exponential value of the number.), where x is the argument, and E is Euler's constant, the base of the natural logarithms.
9. Math.sqrt(x) It will return a square root of a given value.
10. Math.log(x) This method is used to return the natural logarithm (base e) of a number.
11. Math.ceil(x) This method returns the smallest integer, greater than or equal to the given number.
12. Math.floor(x) It returns the greatest integer, smaller than or equal to the given number.
13. Math.round(x) This will round the number passed as a parameter to its nearest integer.
14. Math.random() This method will return a pseudo-random value n , where 0 <= n<1
15. Math.trunc(x) Returns the integer part of the given value and discards its fractional part.
Example:
<!DOCTYPE html>
<html>
<head>
<title> JavaScript Math Object</title>
</head>
<body>
<h2>JavaScript Math Methods</h2>
<p id="demo"></p>
<!-- Script to use math object method -->
<script>
document.getElementById("demo").innerHTML =
"<p><b>Math.abs(-4.7):</b> " + Math.abs(-4.7) + "</p>" +
"<p><b>Math.sin(90 * Math.PI / 180):</b> " +
Math.sin(90 * Math.PI / 180) + "</p>" +
"<p><b>Math.cos(90):</b>" + Math.cos(90) + "</p>" +
"<p><b>Math.tan(90):</b>" + Math.tan(90) + "</p>" +
"<p><b>Math.min(0, 150, 30, 20, -8, -200):</b> " +
Math.min(0, 150, 30, 20, -8, -200) + "</p>" +
"<p><b>Math.max(0, 150, 30, 20, -8, -200):</b> " +
Math.max(0, 150, 30, 20, -8, -200) + "</p>" +
"<p><b>Math.pow(7, 2):</b>" + Math.pow(7, 2) + "</p>" +
"<p><b>Math.exp(30):</b>" + Math.exp(90) + "</p>" +
"<p><b>Math.sqrt(0.5):</b>" + Math.sqrt(90) + "</p>" +
"<p><b>Math.log(10):</b>" + Math.log(10) + "</p>" +
"<p><b>Math.ceil(4.4):</b> " + Math.ceil(4.4) + "</p>" +
"<p><b>Math.floor(4.7):</b> " + Math.floor(4.7) + "</p>" +
"<p><b>Math.round(20.7):</b>" + Math.round(20.7) + "</p>" +
"<p><b>Math.random():</b>" + Math.random() + "</p>" +
"<p><b>Math.trunc(2.77):</b>" + Math.trunc(2.77);
</script>
</body>
</html>
Save the file with the .html extension. Then open it in any browser like Chrome, Firefox... It will give the output as follows;
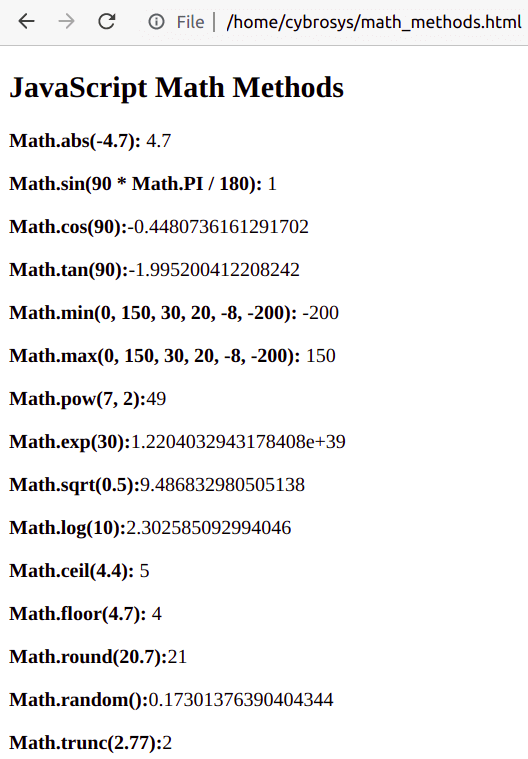
In JavaScript, the Math Object provides different properties and methods to quickly perform a lot of mathematical operations. It’s not a function object. Math object works with the Number type. All the properties and methods of Math are static and can be called by using Math as an object without creating it.