Selenium is one of the most popular web applications for automation and testing. It is a free and open-source tool mainly used for automated testing and validation of web applications in different browsers and operating platforms. The Selenium test scripts can be coded in different programming languages such as C#, Java, Python, etc.
Here, we’ll see in detail how we can automate tests in web applications using selenium and python.
First, let us see what a Selenium Webdriver is.
Webdriver
A Selenium web driver can be considered as a bridge between the web application and the python test script as it is the component that is used to communicate with the web browser. Each webdriver is browser-specific, that is they can only be used for a particular browser and it’s supported by different languages and different platforms.
The Selenium web driver architecture consists of four blocks: Selenium Client Libraries, JSON Wire Protocol, Browser Drivers, and the Browsers. The Selenium Client Libraries consists of the different libraries supported by the language on which we are creating the test script, currently, in our case it is python. The JSON Wire Protocol is conducive for data transfer between the server system and the client. It is a REST API which transfers data between the HTTP servers. The Browser Drivers are used to communicate with the corresponding web browser and the communication is done via HTTP responses. The Browsers are the browsers on which we can use our Webdriver and automate the tests using Selenium. Moreover, it can be used only on browsers to which the Web drivers are available.
The following are the links to download the Selenium Web drivers for common browsers:
Selenium
Now as we have learned about the web drivers, let us see how we can automate and test using the Web drivers and python using Selenium.
Firstly make sure that python and pip are properly installed in your working environment.
To install Selenium in your environment execute the below command.
pip install -U selenium
After the successful installation of the Selenium package, next download the corresponding Webdriver related to the browser on which you are going to automate or test.
Once you have successfully downloaded the Webdriver and installed Selenium, let start by launching the browser in a Head full mode which can be done using the following code.
from selenium import webdriver
DRIVER_PATH = '/path/to/chromedriver'
driver = webdriver.Chrome(executable_path=DRIVER_PATH)
driver.get('https://www.cybrosys.com/')
In the command for the DRIVER_PATH, add the location where you have stored the corresponding web driver for your browser.
Once everything is correct and the script is loaded, we can view that the browser opens up and a message stating that the browser is currently controlled by an automated system.
Similarly, it can be executed in headless mode also where we don’t require any graphical interface as described in the following command.
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
options = Options()
options.headless = True
driver = webdriver.Chrome(options=options,executable_path=DRIVER_PATH)
driver.get("https://www.cybrosys.com/")
driver.quit()
This will load the browser in headless mode.
We can get the HTML code of the current webpage loaded using the command driver.page_source. Similarly, the title and current URL can also be fetched using driver.title and driver.current_url commands respectively. We can also take a screenshot of the current webpage using driver.save_screenshot(test_screenshot.png')
Once everything is loaded and working fine, we can now move to locate elements on the website for which we have several methods to locate elements inside the website. Furthermore, initially inspect the webpage that you want to access (usually Ctrl + Shift + C or on macOS Cmd + Shift + C instead of having to right-click + inspect).
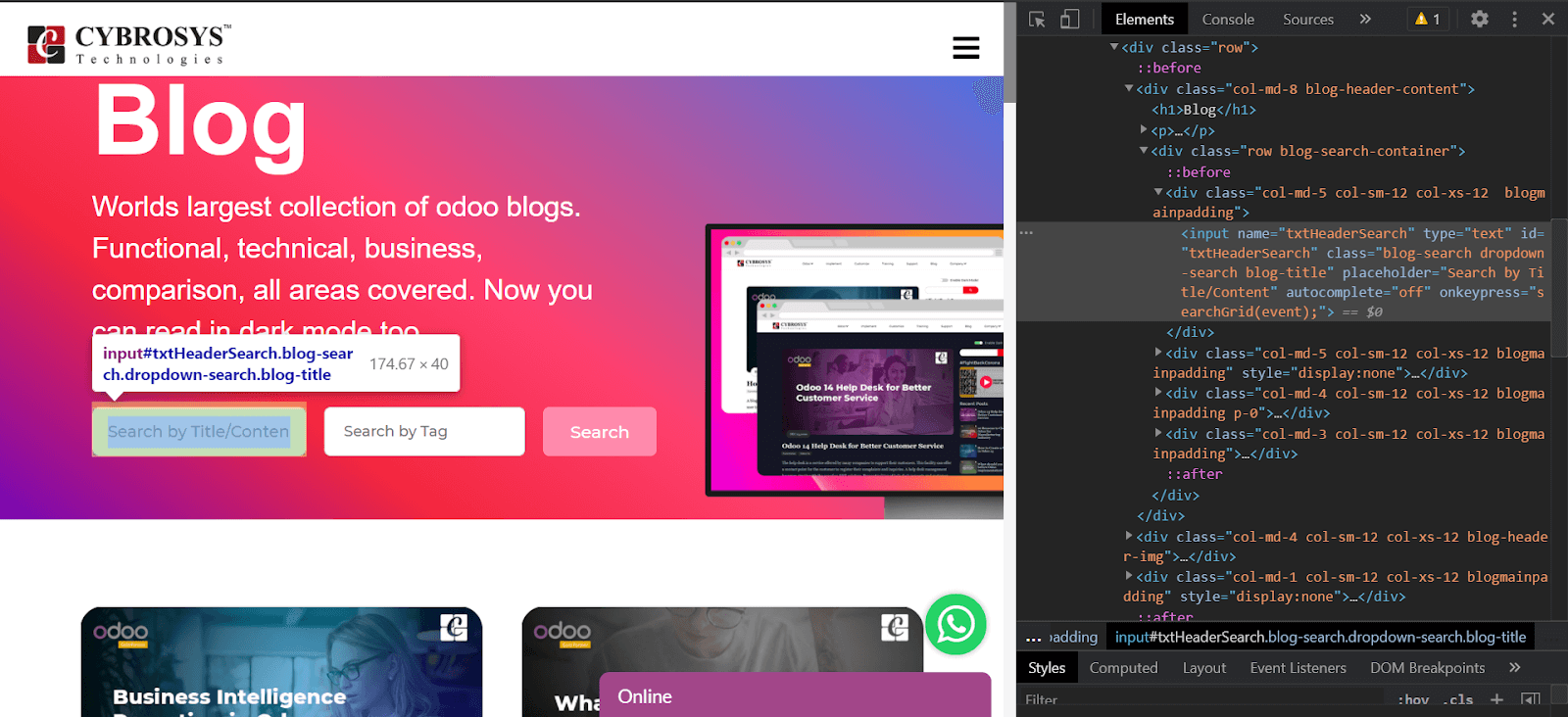
There are several ways by which you can locate the elements some of them are described in the following example.
h1 = driver.find_element_by_name('h1')
div = driver.find_element_by_class_name(‘blog-header-content’)
input= driver.find_element_by_id(‘txtHeaderSearch’)
input= driver.find_element_by_xpath('//*[@id="txtHeaderSearch"]')
In addition, we can get a list of elements matching a particular condition to locate elements. Use find_elements instead of find_element in the above cases.
Now as we have learned how we can locate an element from the website, let us discuss the actions that can be performed on these elements.
Actions on Elements
In order to perform a click action on an element, let’s say search_button, we can call the function by search_button.click()
Similarly, if we want to access the text, we can use h1.text()
Additionally, if we want to send some input to an input field we can use input.send_keys('Value to be passed').
These are some of the basic actions that can be performed using Selenium on the webpage.
Let us see an example where we automate searching a blog from our website.
from selenium import webdriver
blog_topic = input("Enter the blog topic: ")
#initialize the webdriver, the webdriver should be present in the root folder where this script is stored, or else you will have to specify its location.
driver = webdriver.Chrome()
#URL for the web page to be loaded.
driver.get("https://www.cybrosys.com/blog/")
#fetch the input box where we can enter the input to search the blogs
blog_search_box = driver.find_element_by_id("txtHeaderSearch")
#send the blog topic to be searched
blog_search_box.send_keys(blog_topic)
#fetch the search submit button
blog_search_button = driver.find_element_by_id("btnTagsearch")
#call the click event
blog_search_button.click()
driver.quit()
print("Finished")
The above code will load the website and automatically input the values into the search box for searching the blog based on the search text we have provided and automatically submit it by clicking the search button. Thus, automating our process of searching the blog.
Moreover, the testing and automation of large web applications and their functionality can be easily achieved by Selenium in a similar manner saving us a lot of time.
Additionally, we can also use Selenium for web scraping also where we can extract data from the web pages.